Install python
Check does you have python install, open up Terminal and use the following command to check
python3 --version
if the output gives you a version number then you have python installed
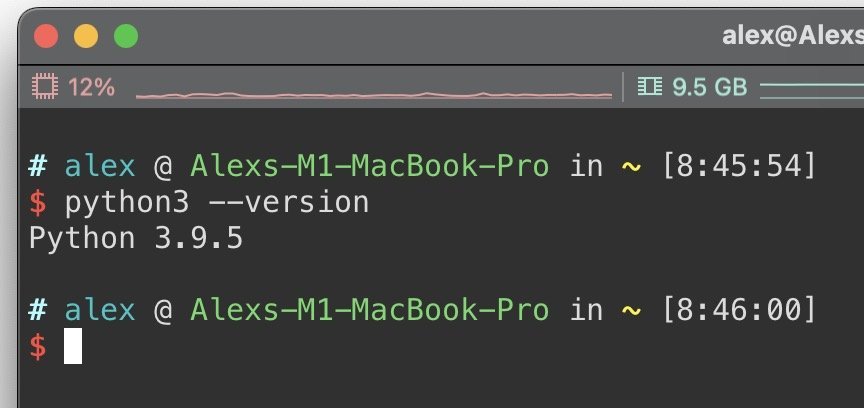
if you get command not found, go to python website to download and install the latest python for your operating system.
Install pip
Check does you have pip install, open up Terminal and use the following command to check
pip --version
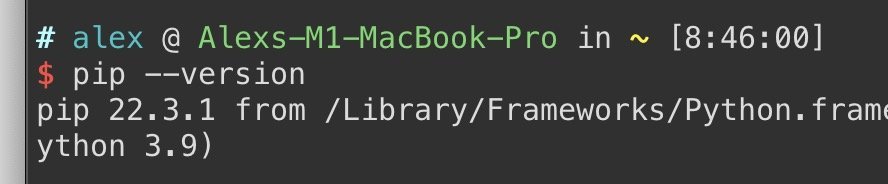
Download setup script
curl https://bootstrap.pypa.io/get-pip.py -o get-pip.py
Run the setup
python3 get-pip.py
Let’s begin
you need to install the python module pynput
pip install pynput
then create a autoclicker.py file
touch autoclicker.py
open up the file with your text editor of your choice and paste in the following code
# importing time and threading
import time
import threading
from pynput.mouse import Controller, Button
# pynput.keyboard is used to watch events of
# keyboard for start and stop of auto-clicker
from pynput.keyboard import Listener, KeyCode
TOGGLE_KEY = KeyCode(char="t")
clicking = False
mouse = Controller()
def clicker():
while True:
if clicking:
mouse.click(Button.left, 1)
time.sleep(0.001)
def toggle_event(key):
if key == TOGGLE_KEY:
global clicking
clicking = not clicking
click_thread = threading.Thread(target=clicker)
click_thread.start()
with Listener(on_press=toggle_event) as listener:
listener.join()
How to run the program
go to terminal and use python3 autoclicker.py to run the python program and use “t” to toggle the auto click function
Buy me a coffee